Mastering Java Background Jobs: Threads, Pools, Virtual Threads, and JobRunr
We’ve all been there. Your web application needs to do something time-consuming; send an email, process an uploaded file, generate a report,.. but making the user wait is simply not an option.
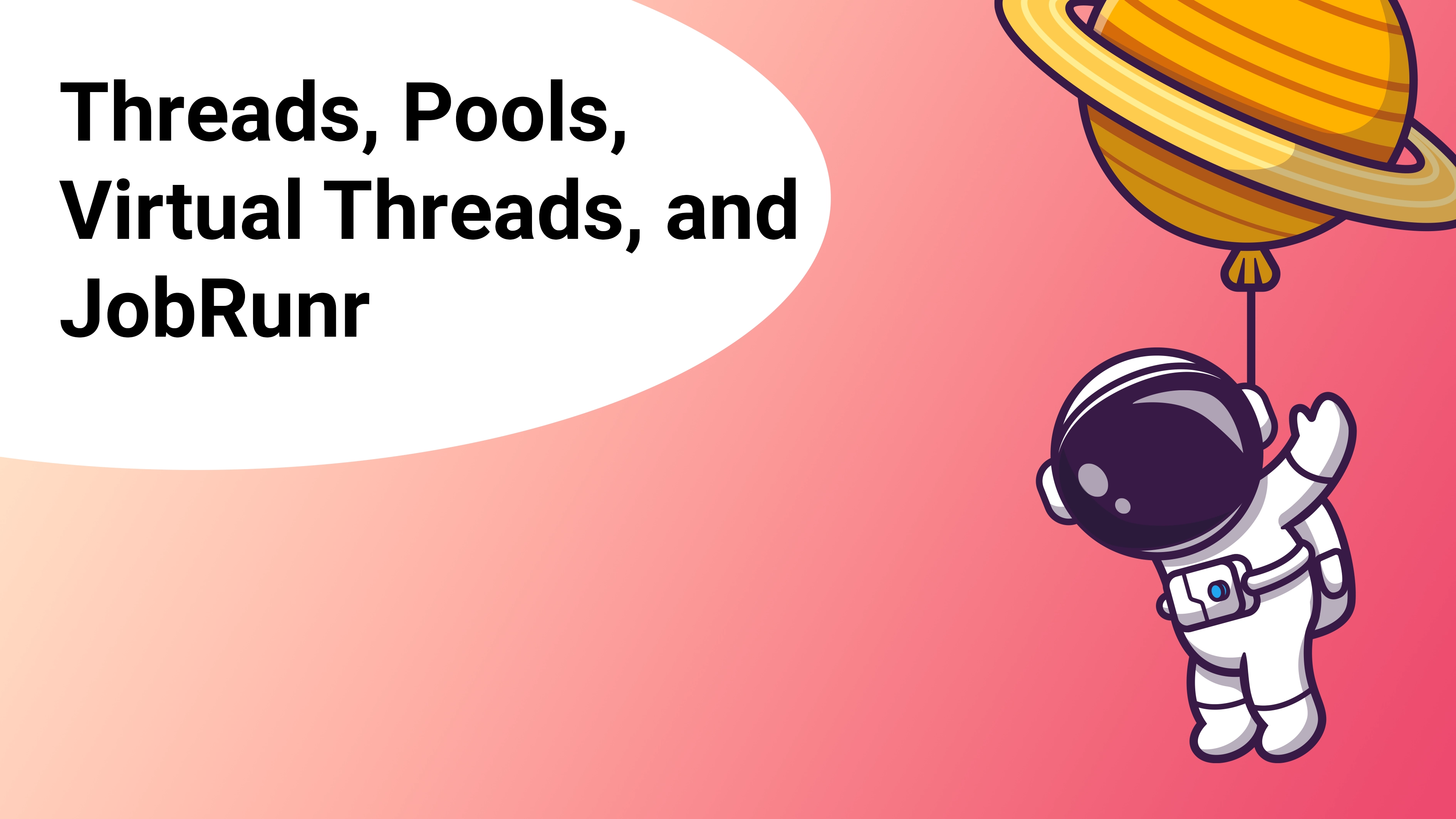
The temptation might be to whip up a quick new Thread().start()
, cross your fingers, and call it a day. Simple, right? Unfortunately, as many of us have learned the hard way in production environments, this naive approach quickly leads to a world of frustration, from crashing servers to silently failing tasks.
So, how do we move beyond basic threads towards robust background job processing in Java? This guide will walk you through the crucial first step: managing concurrency effectively. We’ll compare the dangers of manual threads to structured approaches using Thread Pools and Virtual Threads. We’ll explore common pitfalls and practical solutions in this area, showing how tools like JobRunr are designed to handle this complexity for you.
The Starting Point: Why new Thread()
Isn’t Enough
Let’s quickly revisit why simply spinning up threads manually is problematic for server applications:
- Resource Exhaustion: Remember
OutOfMemoryError
horror stories? Each newThread()
typically creates an OS-level platform thread, consuming significant memory (often 1MB+ for the stack alone). Uncontrolled creation under load is a recipe for crashing your JVM. - Performance Degradation: Even if you don’t run out of memory, having too many platform threads forces your CPU to spend excessive time switching between them (context switching) instead of doing useful work. Your application becomes sluggish as the CPU plays thread traffic cop.
- Lack of Management & Visibility: These rogue threads “are difficult to track because of the lack of visibility”. They can terminate silently on errors, are hard to monitor, and complicate graceful shutdowns. Debugging becomes a nightmare.
Clearly, we need better ways to manage concurrency.
Taming Concurrency: Thread Pools and Virtual Threads
The limitations of manual thread creation lead us to more structured approaches:
1. Thread Pools (ExecutorService
)
This has been the standard Java workhorse for years. Instead of creating a new thread for every task, you use an ExecutorService
to manage a pool of reusable platform threads.
The Executors
utility class provides several factory methods to create different types of pools:
Executors.newFixedThreadPool(int nThreads)
: Creates a pool with a fixed number of threads. If all threads are busy, tasks wait in a queue. This is often preferred for server applications as it provides predictable resource limits.Executors.newCachedThreadPool()
: Creates threads as needed for tasks and reuses threads that have been idle (typically for 60 seconds). While this makes it responsive for handling bursts of short-lived tasks (we are using it for the JobRunr dashboard to handle incoming HTTP traffic), an important caveat is its potential for unbounded growth. Unlike a fixed thread pool, it has no predefined limit and will continue creating threads as long as system resources permit. Under sustained high load, this behavior can lead to resource exhaustion and riskOutOfMemoryError
, much like the problems associated with usingnew Thread()
directly. Therefore, it should be used cautiously in server environments where predictable resource consumption is often preferred.Executors.newSingleThreadExecutor()
: A pool with only one thread, ensuring tasks execute sequentially in the order they are submitted.
Regardless of the type, ExecutorService
provides:
Benefits:
- Resource Control (mostly): Limits the maximum number of active threads (especially fixed pools), helping prevent resource exhaustion.
- Lifecycle Management: Provides mechanisms for starting, stopping, and managing the pool.
- Performance: Reduces the overhead of thread creation/destruction and can limit excessive context switching.
// Example: A fixed-size thread pool
int poolSize = calculateOptimalPoolSize(); // Needs careful consideration!
ExecutorService executor = Executors.newFixedThreadPool(poolSize);
executor.submit(() -> {
System.out.println("Running task in thread pool: " + Thread.currentThread().getName());
// ... your background task logic ...
});
// Remember to shut down the pool gracefully!
// executor.shutdown();
The Catch: Choosing the right type and sizing the pool effectively (for fixed pools) is crucial. Sizing depends heavily on whether your tasks are CPU-bound or I/O-bound. Getting this wrong can lead to bottlenecks. Using cached pools avoids sizing decisions but introduces the risk of unbounded thread creation under load.
2. Virtual Threads (JDK21+)
Virtual threads are a game-changer, especially for I/O-bound workloads. They are lightweight threads managed by the JVM, not directly by the OS.
Benefits:
- Massive Parallelism: You can potentially run millions of virtual threads concurrently with low memory overhead, ideal for tasks that spend most of their time waiting (network calls, DB queries, file I/O).
- Simpler Code: Often allows writing asynchronous code in a more familiar, sequential style.
Caveats:
- They don’t magically make CPU-bound tasks faster; you’re still limited by core count.
- Underlying resource limits (database connections, external API rate limits, memory for task data) still apply.
// Example: Using virtual threads (Java 19+)
try (ExecutorService virtualThreadExecutor = Executors.newVirtualThreadPerTaskExecutor()) {
virtualThreadExecutor.submit(() -> {
System.out.println("Running task in virtual thread: " + Thread.currentThread().isVirtual());
// ... I/O-bound background task logic ...
});
} // Executor shuts down automatically
Managing concurrency effectively is the first step. But even with the right threading model, things can still go wrong…
Let JobRunr Handle the Complexity
As we’ve seen, managing concurrency for background jobs in Java involves careful consideration of thread pools, their types and sizes, or leveraging virtual threads appropriately. Getting it right requires understanding your workload and managing resources diligently.
This is where a dedicated job scheduling framework like JobRunr comes in. Instead of manually configuring and tuning ExecutorService
instances or managing thread lifecycles, JobRunr handles the worker thread management for you. It even defaults to using Virtual Threads on compatible JDKs (21+) for efficient handling of I/O-bound jobs.
Beyond simplifying concurrency, JobRunr provides a complete solution for robust background processing, including:
- Persistence: Saving jobs to a database (SQL/NoSQL) so they survive restarts.
- Automatic Retries: Handling transient failures gracefully.
- Scheduling: Support for fire-and-forget, delayed, and recurring (CRON) jobs.
- Observability: A built-in dashboard for monitoring and managing jobs.
- Distributed Coordination: Ensuring jobs run correctly across multiple application instances.
Next Steps
Take a look at your current background job implementation. Could it be more resilient, scalable, or easier to monitor?
- Explore JobRunr on GitHub.
- Check out the JobRunr Documentation for configuration details, storage options, and features.
- Consider trying JobRunr in your next project to experience how much easier reliable background processing can be!
By applying these principles and leveraging the right tools, you can build Java background job systems that are truly production-ready. Happy coding!