Priority Queues
Priority Queues will make sure your critical business processes finish on-time.
Are you processing millions of jobs? Do you have some high priority jobs that need to finish fast? Use JobRunr priority queues to make sure that critical jobs cut in front of already enqueued jobs.
Note: JobRunr Pro supports up to 5 different priority queues and they can be used together with the load-balancing feature.
Usage
Using the Job Annotation
Using queues could not have been easier thanks to the Job
annotation. Just add it to your service method and specify on which queue you want to run it.
public static final String HighPrioQueue = "HighPrio";
public static final String DefaultQueue = "Default";
public static final String LowPrioQueue = "LowPrio";
public void runJobs() {
BackgroundJob.enqueue(this::startJobOnLowPrioQueue);
BackgroundJob.enqueue(this::startJobOnDefaultQueue);
BackgroundJob.enqueue(this::startJobOnHighPrioQueue);
}
@Job(queue = HighPrioQueue)
public void startJobOnHighPrioQueue() {
System.out.println("This job will bypass all other enqueued jobs.");
}
@Job(queue = DefaultQueue)
public void startJobOnDefaultQueue() {
System.out.println("This job will only bypass jobs on the LowPrioQueue");
}
@Job(queue = LowPrioQueue)
public void startJobOnLowPrioQueue() {
System.out.println("This job will only start when all other jobs on the HighPrioQueue and DefaultQueue are finished.");
}
Using the Job Builder
If you are using the JobBuilder
, queues are also really easy to use and you can even pass the queues at runtime using a variable:
public void startJobOnQueue(JobQueue jobQueue) { // JobQueue can be an enum value
jobScheduler.create(aJob()
.withQueue(jobQueue)
.withDetails(() -> System.out.println("This job will start on the given queue"));
}
Configuration
Configuration is easy, both in the fluent api and using Spring configuration:
Fluent Api
Using the fluent API, first specify all the queues as Strings (or string constants) and then continue the configuration as normal.
JobRunrPro
.configure()
.useQueues(DefaultQueue, HighPrioQueue, DefaultQueue, LowPrioQueue)
...
Framework configuration using property file
For the Spring / Micronaut / Quarkus, you can just define the queues in your configuration file. Below you can find the example for Spring.
org.jobrunr.queues.default-queue-name=Default
org.jobrunr.queues.names=HighPrio, Default, LowPrio
#org.jobrunr.queues.from-enum=org.jobrunr.examples.services.JobRunrQueues # you can also pass the fully qualified name to an enum but due to the Java compiler, enums van not be used in an annotation
Framework configuration using beans
You can also configure the Queues using beans by means of an extra bean of type Queues
. This bean must then be passed to the JobRunrDashboardWebServer
and the JobScheduler
.
@Bean
public Queues queues() {
return new Queues(defaultQueue, queues);
}
@Bean
public JobRunrDashboardWebServer dashboardWebServer(StorageProvider storageProvider, JsonMapper jsonMapper, Queues queues) {
final JobRunrDashboardWebServer jobRunrDashboardWebServer = new JobRunrDashboardWebServer(storageProvider, jsonMapper, queues);
jobRunrDashboardWebServer.start();
return jobRunrDashboardWebServer;
}
@Bean
public JobScheduler jobScheduler(StorageProvider storageProvider, Queues queues) {
JobScheduler jobScheduler = new JobScheduler(storageProvider, queues);
BackgroundJob.setJobScheduler(jobScheduler);
return jobScheduler;
}
Dashboard
The Pro version of JobRunr comes with an enhanced dashboard that shows you the different queues.
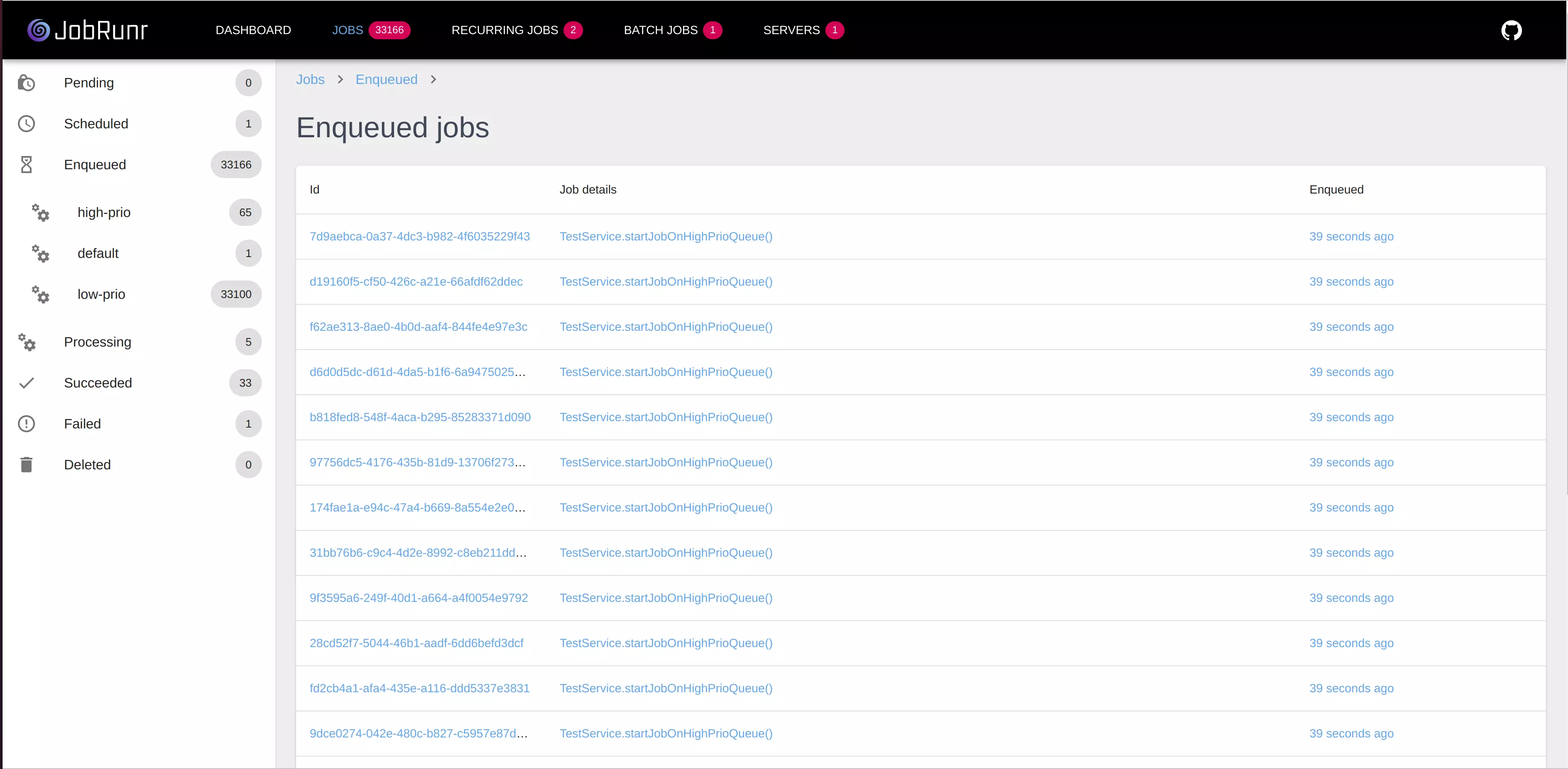